There are times when you need to return a large text from a web service. The large text will then need to be handled by the recipient. In order to reduce the size of the message you can combine two simple techniques:
- Compress the string value with a compression algorithm, such as BZip2
- Base64 encode the resulting byte array
You will be able to send the base 64 encoded compressed string over the wire.
You’ll need to import the following NuGet package to use BZip2:
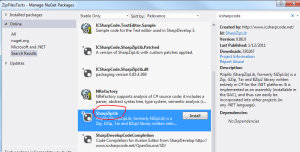
This is how you can compress a string and base 64 encode it:
string largeUncompressedText = "<root><value size=\"xxl\">This is a large text</value></root>";
string largeCompressedText = string.Empty;
using (MemoryStream source = new MemoryStream(Encoding.UTF8.GetBytes(largeUncompressedText)))
{
using (MemoryStream target = new MemoryStream())
{
BZip2.Compress(source, target, true, 4096);
byte[] targetByteArray = target.ToArray();
largeCompressedText = Convert.ToBase64String(targetByteArray);
}
}
The variable “largeCompressedText” can be sent to a listener who will be able to read it as follows:
byte[] largeCompressedTextAsBytes = Convert.FromBase64String(largeCompressedText);
using (MemoryStream source = new MemoryStream(largeCompressedTextAsBytes))
{
using (MemoryStream target = new MemoryStream())
{
BZip2.Decompress(source, target, true);
string uncompressedString = Encoding.UTF8.GetString(target.ToArray());
Console.WriteLine(uncompressedString);
}
}
The example is not perfect in a sense that largeCompressedText will be bigger than the actual source string but you’ll see the benefits with much larger texts.
View all posts related to string and text operations here.